Chapter 4: Getting comment details
In Chapter 4 we used get_selected_marks
to get the user’s selection from the Tableau Dashboard. In this chapter, we’ll save the key fields we need to allow users to make a comment.
We want to get the name of the state and the profit from the dashboard, which are both contained in user_selection
. Let’s add some code to our form that saves this information to form attributes:
1from ._anvil_designer import MainTemplate
2from anvil import *
3from anvil.tables import app_tables
4from anvil import tableau
5
6from trexjacket.api import get_dashboard
7dashboard = get_dashboard()
8
9class Main(MainTemplate):
10 def __init__(self, **properties):
11 self.state_name = None
12 self.profit = None
13 self.logged_in_user = None
14 self.init_components(**properties)
15 dashboard.register_event_handler('selection_changed', self.selection_changed_event_handler)
16
17 def selection_changed_event_handler(self, event):
18 user_selection = event.worksheet.get_selected_marks()
19
20 if len(user_selection) == 0:
21 self.state_name = None
22 self.profit = None
23 self.logged_in_user = None
24 else:
25 record = user_selection[0]
26 self.state_name = record['State']
27 self.profit = record['AGG(Profit Ratio)']
28 self.logged_in_user = record['logged_in_user']
29
30 msg = f"User: {self.logged_in_user}\nState: {self.state_name}\nProfit Ratio: {self.profit}"
31 Notification(msg).show()
Once you’ve added this code in the code pane of Main
, reload the extension inside Tableau and click on a few states. You should see the selection appearing as a popup in your extension!
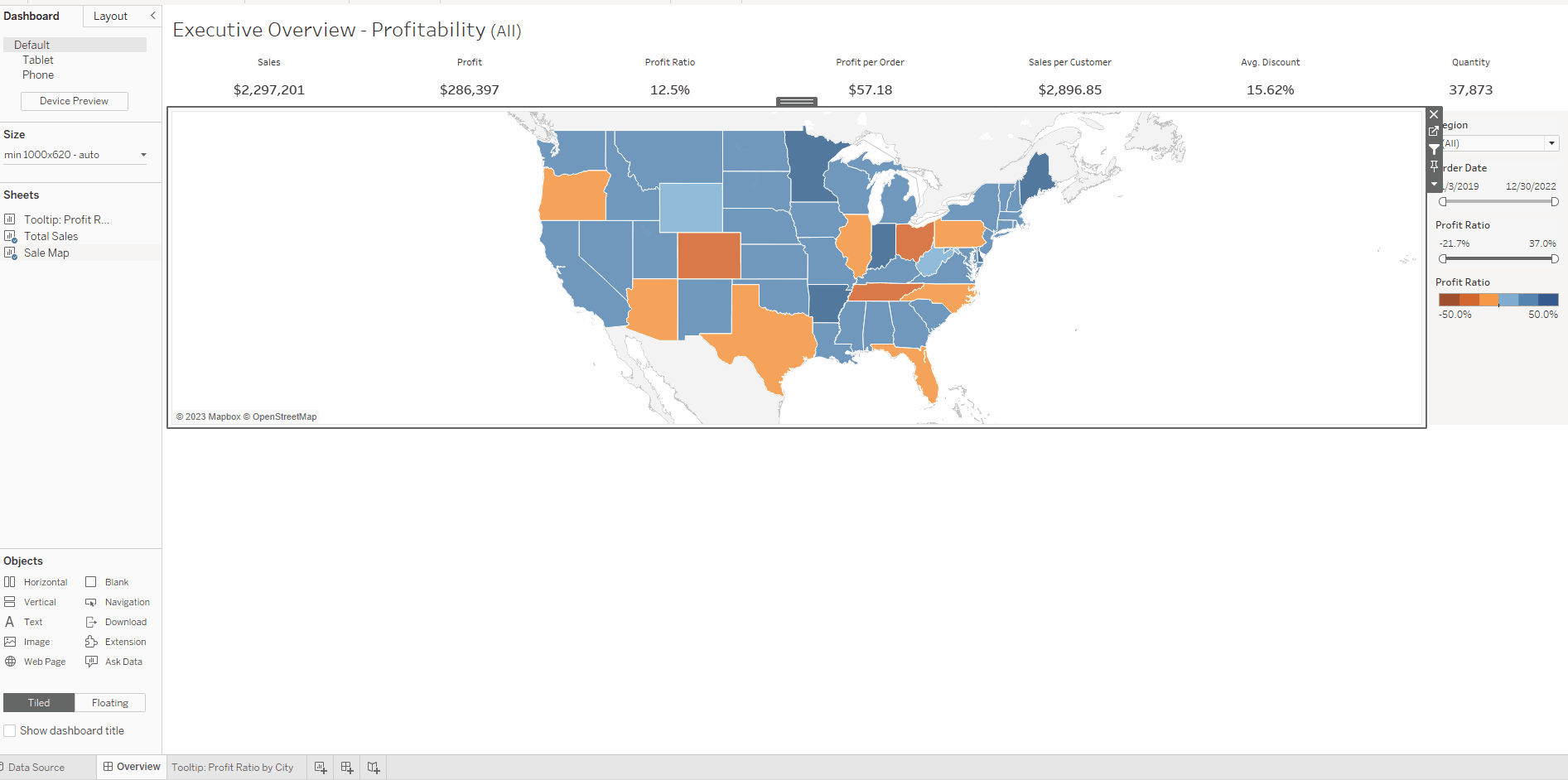
In the next chapter we’ll add the functionality for a user to add their comment.